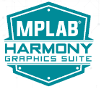 |
MPLABĀ® Harmony Graphics Suite
|
Go to the documentation of this file.
43 #ifndef LEGATO_BUTTON_H
44 #define LEGATO_BUTTON_H
48 #if LE_BUTTON_WIDGET_ENABLED == 1
72 typedef enum leButtonState
76 LE_BUTTON_STATE_TOGGLED
83 typedef struct leButtonWidget leButtonWidget;
96 typedef void (*leButtonWidget_PressedEvent)(leButtonWidget*);
109 typedef void (*leButtonWidget_ReleasedEvent)(leButtonWidget*);
118 typedef struct leButtonWidget leButtonWidget;
120 #define LE_BUTTONWIDGET_VTABLE(THIS_TYPE) \
121 LE_WIDGET_VTABLE(THIS_TYPE) \
123 leBool (*getToggleable)(const THIS_TYPE* _this); \
124 leResult (*setToggleable)(THIS_TYPE* _this, leBool toggleable); \
125 leBool (*getPressed)(const THIS_TYPE* _this); \
126 leResult (*setPressed)(THIS_TYPE* _this, leBool pressed); \
127 leString* (*getString)(const THIS_TYPE* _this); \
128 leResult (*setString)(THIS_TYPE* _this, const leString* str); \
129 leImage* (*getPressedImage)(const THIS_TYPE* _this); \
130 leResult (*setPressedImage)(THIS_TYPE* _this, leImage* img); \
131 leImage* (*getReleasedImage)(const THIS_TYPE* _this); \
132 leResult (*setReleasedImage)(THIS_TYPE* _this, leImage* img); \
133 leRelativePosition (*getImagePosition)(const THIS_TYPE* _this); \
134 leResult (*setImagePosition)(THIS_TYPE* _this, leRelativePosition pos); \
135 uint32_t (*getImageMargin)(const THIS_TYPE* _this); \
136 leResult (*setImageMargin)(THIS_TYPE* _this, uint32_t mg); \
137 int32_t (*getPressedOffset)(const THIS_TYPE* _this); \
138 leResult (*setPressedOffset)(THIS_TYPE* _this, int32_t offs); \
139 leButtonWidget_PressedEvent (*getPressedEventCallback)(const THIS_TYPE* _this); \
140 leResult (*setPressedEventCallback)(THIS_TYPE* _this, leButtonWidget_PressedEvent cb); \
141 leButtonWidget_ReleasedEvent (*getReleasedEventCallback)(const THIS_TYPE* _this); \
142 leResult (*setReleasedEventCallback)(THIS_TYPE* _this, leButtonWidget_ReleasedEvent cb); \
145 typedef struct leButtonWidgetVTable
147 LE_BUTTONWIDGET_VTABLE(leButtonWidget)
148 } leButtonWidgetVTable;
175 typedef struct leButtonWidget
179 const leButtonWidgetVTable* fn;
190 uint32_t imageMargin;
191 int32_t pressedOffset;
193 leButtonWidget_PressedEvent pressedEvent;
194 leButtonWidget_ReleasedEvent releasedEvent;
214 LIB_EXPORT leButtonWidget* leButtonWidget_New();
226 LIB_EXPORT
void leButtonWidget_Constructor(leButtonWidget* wgt);
229 #define THIS_TYPE struct leWidget
241 virtual leBool getToggleable(
const leButtonWidget* _this);
256 virtual leResult setToggleable(leButtonWidget* _this,
269 virtual leBool getPressed(
const leButtonWidget* _this);
284 virtual leResult setPressed(leButtonWidget* _this,
298 virtual leString* getString(
const leButtonWidget* _this);
314 virtual leResult setString(leButtonWidget* _this,
327 virtual leImage* getPressedImage(
const leButtonWidget* _this);
343 virtual leResult setPressedImage(leButtonWidget* _this,
357 virtual leImage* getReleasedImage(
const leButtonWidget* _this);
372 virtual leResult setReleasedImage(leButtonWidget* _this,
402 virtual leResult setImagePosition(leButtonWidget* _this,
415 virtual uint32_t getImageMargin(
const leButtonWidget* _this);
430 virtual leResult setImageMargin(leButtonWidget* _this,
445 virtual int32_t getPressedOffset(
const leButtonWidget* _this);
461 virtual leResult setPressedOffset(leButtonWidget* _this,
474 virtual leButtonWidget_PressedEvent getPressedEventCallback(
const leButtonWidget* _this);
489 virtual leResult setPressedEventCallback(leButtonWidget* _this,
490 leButtonWidget_PressedEvent cb);
502 virtual leButtonWidget_ReleasedEvent getReleasedEventCallback(
const leButtonWidget* _this);
518 virtual leResult setReleasedEventCallback(leButtonWidget* _this,
519 leButtonWidget_ReleasedEvent cb);
Error functions, macros and definitions.
leResult
This enum represents function call results.
Definition: legato_common.h:134
This struct represents a rectangle.
Definition: legato_common.h:405
Image functions and defintions.
Memory functions and definitions.
Definition: legato_image.h:180
@ LE_HALIGN_CENTER
Definition: legato_common.h:208
This struct represents a string.
Definition: legato_string.h:108
leBool
This enum represents booleans.
Definition: legato_common.h:157
virtual uint32_t length(const leString *_this)
Get length of the string.
@ LE_FALSE
Definition: legato_common.h:158
LIB_EXPORT leBool leRectContainsPoint(const leRect *rect, const lePoint *point)
Determines if a point is inside a rectangle.
Definition: legato_rect.c:31
General internal utilities for the library.
Fixed string functions and definitions.
This struct represents a c-style string render request.
Definition: legato_string_renderer.h:128
@ LE_RELATIVE_POSITION_RIGHTOF
Definition: legato_common.h:317
@ LE_HALIGN_LEFT
Definition: legato_common.h:207
String utility functions and definitions.
void leUtils_RectToScreenSpace(const leWidget *widget, leRect *rect)
Convert rectangle from widget local space to screen space.
Definition: legato_utils.c:151
The header file joins all header files used in the graphics object library.
void leUtils_ClipRectToParent(const leWidget *widget, leRect *rect)
Clips a rectangle to the parent of a widge.
Definition: legato_utils.c:112
LIB_EXPORT void leRectClip(const leRect *l_rect, const leRect *r_rect, leRect *result)
Clips a rectangle to the space of another rectangle.
Definition: legato_rect.c:122
void * leArray_Get(const leArray *arr, uint32_t idx)
Get entry at index.
Definition: legato_array.c:224
@ LE_RELATIVE_POSITION_LEFTOF
Definition: legato_common.h:314
leResult leStringRenderer_DrawCString(leCStringRenderRequest *req)
Draw leChar string.
Definition: legato_string_renderer.c:297
leColor leScheme_GetRenderColor(const leScheme *schm, leSchemeColor clr)
Gets a scheme render color for the current layer color mode.
Definition: legato_scheme.c:68
leRelativePosition
This enum represents the relative position modes for objects.
Definition: legato_common.h:313
@ LE_TRUE
Definition: legato_common.h:159
Common macros and definitions used by Legato.
const leStringVTable * fn
Definition: legato_string.h:109
LIB_EXPORT leResult leStringUtils_GetRectCStr(const char *str, const leFont *font, leRect *rect)
Gets the bounding rectangle for a C-style string.
Definition: legato_stringutils.c:128
This structure represents a integer Cartesian point.
Definition: legato_common.h:357